Brent Simmons, the original developer of MarsEdit and NetNewsWire, is building a new feed reader app called Evergreen:
Evergreen is an open source, productivity-style feed reader for Macs.
It’s at a very early stage – we use it, but we don’t expect other people to use it yet.
I’ve never been one to shy away from early-stage software, so of course I ran to the GitHub project page, cloned the repository, and built it immediately on my own Mac.
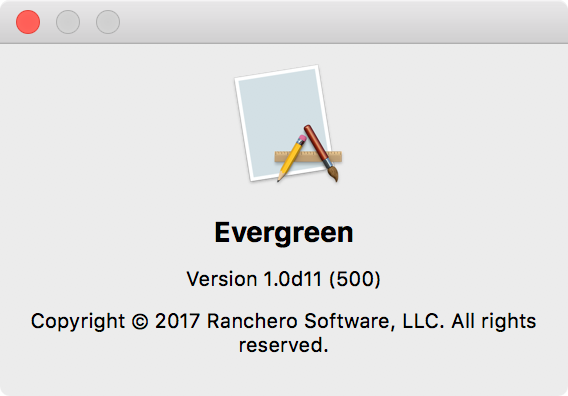
Ahh, the tell-tale sign of a young app: the generic about box. Personally, I like to give apps-in-progress an icon, even if only a placeholder image, as soon as possible. It occurred to me that Apple has done the favor of providing a pretty-darned-suitable image for “Evergreen” in the form of its Emoji glyph of the same name:
🌲
Since I have the source code right here, why don’t I render that tree at a large size in a graphics app, resize it to a million different resolutions, bundle it up and check it in to the Evergreen source base?
Because that’s not nearly as fun as doing it in code. I dove into the Evergreen application delegate class, adding the following function:
private func evergreenImage() -> NSImage? {
var image: NSImage? = nil
let imageWidth = 1024
let imageHeight = 1024
let imageSize = NSMakeSize(CGFloat(imageWidth), CGFloat(imageHeight))
if let drawingContext = CGContext(data: nil, width: imageWidth, height: imageHeight, bitsPerComponent: 8, bytesPerRow: 0, space: CGColorSpaceCreateDeviceRGB(), bitmapInfo: CGImageAlphaInfo.premultipliedFirst.rawValue) {
let graphicsContext = NSGraphicsContext(cgContext: drawingContext, flipped: false)
NSGraphicsContext.saveGraphicsState()
NSGraphicsContext.setCurrent(graphicsContext)
let targetRect = NSRect(origin: NSZeroPoint, size: imageSize)
NSString(string: "🌲").draw(in: targetRect, withAttributes: [NSFontAttributeName: NSFont.systemFont(ofSize: 1000)])
NSGraphicsContext.restoreGraphicsState()
if let coreImage = drawingContext.makeImage() {
image = NSImage(cgImage: coreImage, size: imageSize)
}
}
return image
}
In summary this code: creates a CoreGraphics drawing context, renders a huge evergreen Emoji glyph into it, and creates an NSImage out of it.
Then from the “applicationDidFinishLaunching()” function:
if let appIconImage = evergreenImage() {
appIconImage.setName("NSApplicationIcon")
NSApplication.shared().applicationIconImage = appIconImage
}
Give the newly created image the canonical name, used by AppKit, for looking up the application icon, and immediately change the application’s icon image to reflect the new value. It worked a treat:
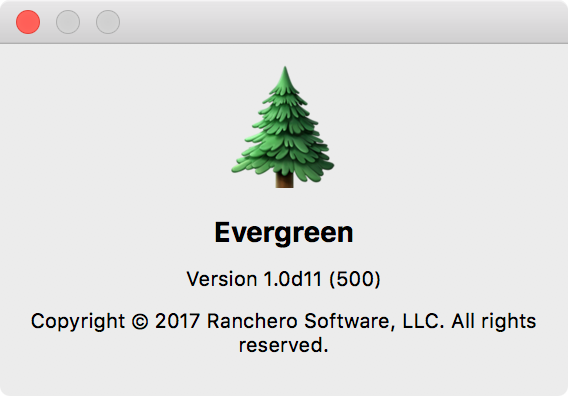
In programming there is usually a hard way, an easy way, and a fun way. Be sure to take the third option as often as possible.